Catalog of Observed Gravitational-wave Mergers¶
Information about the growing catalog of gravitational-wave mergers can be
accessed through the pycbc.catalog
package.
Which mergers do we have information about?¶
import pycbc.catalog
c = pycbc.catalog.Catalog(source='gwtc-2')
# Names of mergers in the catalog
print(c.names)
# Approximate GPS time of the mergers
print([c[m].time for m in c])
$ python ../examples/catalog/what.py
dict_keys(['GW190408_181802-v1', 'GW190412-v3', 'GW190413_052954-v1', 'GW190413_134308-v1', 'GW190421_213856-v1', 'GW190424_180648-v1', 'GW190425-v2', 'GW190426_152155-v1', 'GW190503_185404-v1', 'GW190512_180714-v1', 'GW190513_205428-v1', 'GW190514_065416-v1', 'GW190517_055101-v1', 'GW190519_153544-v1', 'GW190521-v3', 'GW190521_074359-v1', 'GW190527_092055-v1', 'GW190602_175927-v1', 'GW190620_030421-v1', 'GW190630_185205-v1', 'GW190701_203306-v1', 'GW190706_222641-v1', 'GW190707_093326-v1', 'GW190708_232457-v1', 'GW190719_215514-v1', 'GW190720_000836-v1', 'GW190727_060333-v1', 'GW190728_064510-v1', 'GW190731_140936-v1', 'GW190803_022701-v1', 'GW190814-v2', 'GW190828_063405-v1', 'GW190828_065509-v1', 'GW190909_114149-v1', 'GW190910_112807-v1', 'GW190915_235702-v1', 'GW190924_021846-v1', 'GW190929_012149-v1', 'GW190930_133541-v1'])
[1238782700.3, 1239082262.2, 1239168612.5, 1239198206.7, 1239917954.3, 1240164426.1, 1240215503.0, 1240327333.3, 1240944862.3, 1241719652.4, 1241816086.8, 1241852074.8, 1242107479.8, 1242315362.4, 1242442967.4, 1242459857.5, 1242984073.8, 1243533585.1, 1245035079.3, 1245955943.2, 1246048404.6, 1246487219.3, 1246527224.2, 1246663515.4, 1247608532.9, 1247616534.7, 1248242632.0, 1248331528.5, 1248617394.6, 1248834439.9, 1249852257.0, 1251009263.8, 1251010527.9, 1252064527.7, 1252150105.3, 1252627040.7, 1253326744.8, 1253755327.5, 1253885759.2]
Plotting some key statistics from the catalog¶
import matplotlib.pyplot as pp
import pycbc.catalog
c = pycbc.catalog.Catalog(source='gwtc-2')
mchirp, elow, ehigh = c.median1d('mchirp', return_errors=True)
spin = c.median1d('chi_eff')
pp.errorbar(mchirp, spin, xerr=[-elow, ehigh], fmt='o', markersize=7)
pp.xlabel('Chirp Mass')
pp.xscale('log')
pp.ylabel('Effective Spin')
pp.show()
(Source code, png, hires.png, pdf)
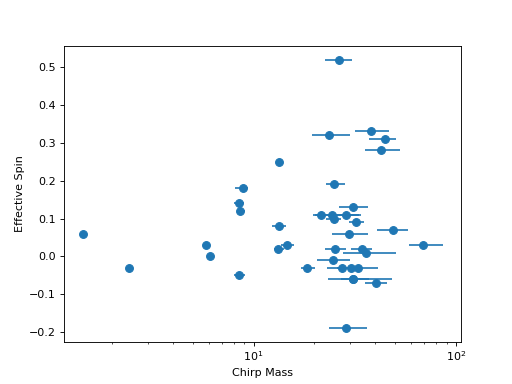
Accessing data around each event¶
Data around each event can also be easily accessed for any detector.
import matplotlib.pyplot as pp
import pycbc.catalog
m = pycbc.catalog.Merger("GW170817", source='gwtc-1')
fig, axs = pp.subplots(2, 1, sharex=True, sharey=True)
for ifo, ax in zip(["L1", "H1"], axs):
pp.sca(ax)
pp.title(ifo)
# Retreive data around the BNS merger
ts = m.strain(ifo).time_slice(m.time - 15, m.time + 6)
# Whiten the data with a 4s filter
white = ts.whiten(4, 4)
times, freqs, power = white.qtransform(.01, logfsteps=200,
qrange=(110, 110),
frange=(20, 512))
pp.pcolormesh(times, freqs, power**0.5, vmax=5)
pp.yscale('log')
pp.ylabel("Frequency (Hz)")
pp.xlabel("Time (s)")
pp.show()
(Source code, png, hires.png, pdf)
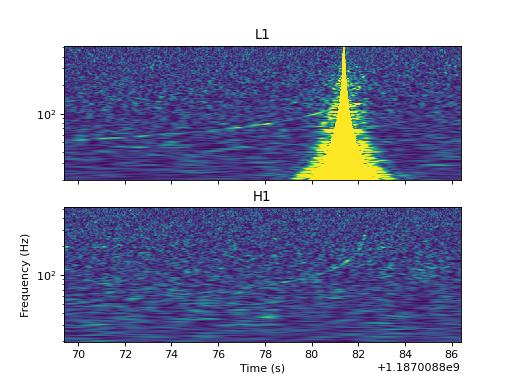